I want register JavaScript events to toggle CSS opacity on selectable images.
For example, given a div with a list of images like the following,
<div id="foo_images"> <img src="foo.jpg" /> <img src="bar.jpg" /> <img src="baz.jpg" /> ... </div>
I would like these images to be 50% transparent but 80% visible during a mouseover. I would also like these images to be selectable, i.e., an image should become fully visible after clicking it.
I would like to do this in JavaScript without having to modify the html image list.
We could add transparency in the stylesheet, as well as the mouseover effect, e.g.,
#foo_images img { filter: alpha(opacity=50); opacity: 0.5; } #foo_images img:hover { filter: alpha(opacity=80); opacity: 0.8; }
In practice, it is highly recommended to keep all presentation elements in the stylesheet, but for the sake of example we can adjust an objects transparency using JavaScript, e.g.,
//set obj transparency to 50% obj.style.opacity = 0.5; //non-IE browsers obj.style.filter = 'alpha(opacity=50)'; //IE
Using this approach, we can register events with JavaScript so that the transparency will change as the cursor hovers over, e.g.,
obj.onmouseover = function() { obj.style.opacity = 0.8; obj.style.filter = 'alpha(opacity=80)'; } obj.onmouseout = function() { obj.style.opacity = 0.5; obj.style.filter = 'alpha(opacity=50)'; }
This would obviously be a lot of repeated code so we can use JavaScript closures, e.g.,
function opacityC(obj, value) {return function() { obj.style.opacity = value/100; obj.style.filter = 'alpha(opacity=' + value + ')'; }} obj.onmouseover = opacityC(obj,80); obj.onmouseout = opacityC(obj,50);
Next, to make the images selectable we can assign onclick events. We’ll need to toggle between selected and not-selected, so we’ll dynamically add a selected attribute to the image object. We can use the same closure approach to assign a function to the onclick event, e.g.,
function toggleSelectC(obj) {return function() { if (obj.selected != 'selected') { opacityC(obj, 100)(); obj.onmouseout = opacityC(obj, 100); obj.style.border = '1px solid black'; obj.selected = 'selected'; } else { obj.onmouseout = opacityC(obj, 50); obj.style.border = '1px solid white'; obj.selected = ''; } }} image1.onclick = toggleSelectC(image1); image2.onclick = toggleSelectC(image2);
Finally, we will loop through all of the images in the div, set the initial transparency and add event handlers, e.g.,
var obj = document.getElementById('foo_images'); var images = obj.getElementsByTagName("img"); for (var i = 0; i < images.length; i++) { var img = images[i]; opacityC(img,50)(); img.onmouseover = opacityC(img, 80); img.onmouseout = opacityC(img, 50); img.onclick = toggleSelectC(img); }
Putting this all together, you would have the following HTML and JavaScript:
<div id="foo_images"> <img src="foo.jpg" /> <img src="bar.jpg" /> <img src="baz.jpg" /> </div> <script type="text/javascript" defer><!-- /** * closure to adjust an objects transparency */ function opacityC(obj, value) {return function() { obj.style.opacity = value/100; obj.style.filter = 'alpha(opacity=' + value + ')'; }} /** * closure to toggle selected/non-selected style */ function toggleSelectC(obj) {return function() { if (obj.selected != 'selected') { opacityC(obj, 100)(); obj.onmouseout = opacityC(obj, 100); obj.style.border = '1px solid black'; obj.selected = 'selected'; } else { opacityC(obj, 50)(); obj.onmouseout = opacityC(obj, 50); obj.style.border = '1px solid white'; obj.selected = ''; } }} /** * register all selectable images */ function registerSelectableImages(obj) { var images = obj.getElementsByTagName("img"); for (var i = 0; i < images.length; i++) { var img = images[i]; opacityC(img,50)(); img.style.border = '1px solid white'; img.onmouseover = opacityC(img, 80); img.onmouseout = opacityC(img, 50); img.onclick = toggleSelectC(img); } } registerSelectableImages(document.getElementById('foo_images')); --></script>
Applying the above code to the three sample images will produce the following results:
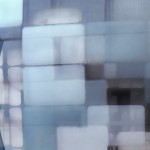
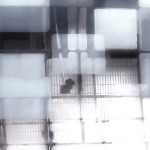
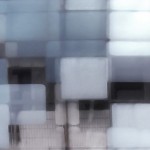